6.2. Working with Matrices¶
We introduced both vectors and matrices in Vectors and Matrices in MATLAB and discussed how to create them in MATLAB. Here, we present mathematical operations on matrices and review important properties of matrices.
6.2.1. Matrix Math¶
6.2.1.1. Addition and Subtraction¶
Addition and subtraction of matrices is performed element-wise.
Scalar and element-wise operations between matrices work the same as with vectors. Of course, the size of both matrices must be the same ( Element-wise Arithmetic).
6.2.1.2. Matrix Multiplication¶
Multiplication of a vector by a matrix and multiplication of two matrices are
common linear algebra operations. The former is used in the expression of a
system of linear equations of the form .
Points and vectors can be moved, scaled, rotated, or skewed when multiplied by
a matrix. This has application to physical geometry and also to pixels in an
image. Two primary uses of matrix–to–matrix multiplication are explored in
later sections. First, we discuss geometric transformation matrices in
Geometric Transforms. The transformation matrices are multiplied with moveable
points to determine new coordinate locations. Multiplication of the
transformation matrices is also used to combine transformations. Secondly, we
will cover several matrix factorings later in this chapter and in the next
chapter, such as
,
,
, and
. Each matrix factoring
facilitates solving particular problems. It is matrix multiplication that
verifies that the product of the factors yields the original matrix.
Here is an example showing both the product of matrix factors and how
multiplying a vector by a matrix scales and rotates vectors and points.
We begin with a vector on the axis and a matrix that rotates
points and vectors
radians (). Rotation matrices are
discussed in Rotation of a Point. We multiply the rotation matrix by
two so that it also scales the vector. Then we use the singular value
decomposition [1] (SVD), which is later described in
Singular Value Decomposition (SVD). With matrix multiplication, we see that the product of the
factors is the same as our original matrix. Then figure
Fig. 6.11 shows that successive multiplications by the
factors rotate, scale, and again rotate the vector to yield the same
result as multiplication by the original scaling and rotating matrix
(
). [2]
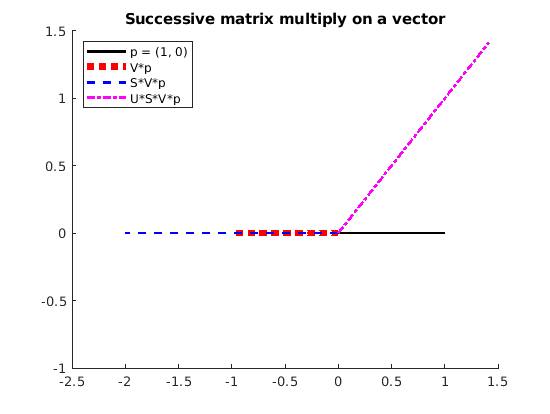
Fig. 6.11 The first matrix multiplication rotates the vector. The second
multiplication scales the vector. The third multiplication rotates
the vector again. The three successive multiplications achieve the
same result as multiplying the vector by .
p = [1;0];
R = [0.7071 -0.7071;
0.7071 0.7071];
[U,S,V] = svd(2*R);
p1 = V'*p; % (-1, 0)
p2 = S*p1; % (-2, 0)
p3 = U*p2; % (1.4, 1.4)
The elements of a matrix product are found by inner products. To complete
the inner products, the number of columns of the left matrix must match the
number of rows in the right matrix. We say that the inner dimensions of the
two matrices must agree. As shown in Size of Matrix Multiplications, A
matrix may be multiplied by a
matrix
to yield a
matrix. The size of the product matrix is the
outer dimensions of the matrices.
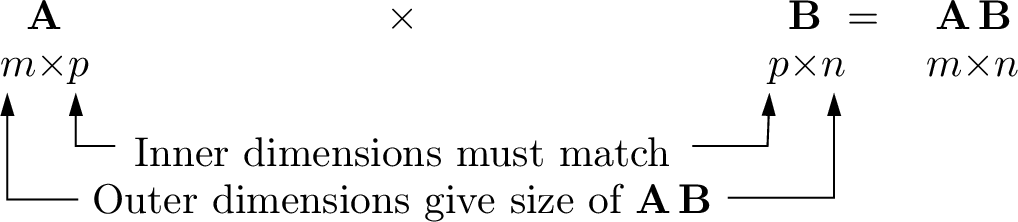
First Matrix | Second Matrix | Output Matrix |
---|---|---|
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
![]() |
With regard to matrix multiplication, we will view vectors as a matrix
with only one column (size ). Multiplication of a
matrix by a vector is defined as either the linear combination of the
columns of the matrix with the vector elements, or as the inner product
between the rows of the left matrix and the column vector.
The individual values of a matrix product are
calculated as inner products between the rows of the left matrix
(
) and the columns of the right matrix (
).
For matrices:
6.2.1.3. Matrix Multiplication Properties¶
Matrix multiplication is associative:
.
In general, matrix multiplication is NOT commutative even for square matrices: (
). The more obvious exceptions to this are multiplication by the identity matrix and an inverse matrix. Note below that the two products of the same matrices are not the same.
does not imply that
. For example, consider matrices:
The Outer Product View of Matrix Multiplication
We normally think of matrix multiplication as finding each term of the matrix product from the sum of products between the rows of the left matrix and the columns of the right matrix. This is the inner product view of matrix multiplication. But there is also an outer product view that is columns times rows.
Each column of a
matrix
multiplies
, the
row of a
matrix. The product
is
an
matrix of rank one formed from the outer
product of the two vectors. The final product
is the
sum of the rank-one matrices.
Outer product matrix multiplication is not how we usually multiply two matrices. Rather, it gives an alternative perspective of what matrix multiplication accomplishes. This perspective will be useful later when we learn about the singular value decomposition.
6.2.1.4. Matrix Division¶
Matrix division, in the sense of scalar division, is not defined for
matrices. Multiplication by the inverse of a matrix accomplishes the
same objective as division does in scalar arithmetic. MATLAB also has
two alternative operators to the matrix inverse that act as one might
expect from division operators, left-divide (\
) and right-divide
(/
); however, they are more complicated than simple division. As
shown in
MATLAB’s Matrix Division Operators, they are used to solve systems of linear equations as described in Systems of Linear Equations.
\ | left-divide | A*x = b |
x = A\b |
x = inv(A)*b |
/ | right-divide | x*A = b |
x = b/A |
x = b*inv(A) |
6.2.2. Special Matrices¶
-
Zero Matrix
A zero vector or matrix contains all zero elements and is denoted as
.
-
Diagonal Matrix
A diagonal matrix has zeros everywhere except on the main diagonal, which is the elements where the row index and column index are the same. Diagonal matrices are usually square (same number of rows and columns), but they may be rectangular with extra rows or columns of zeros.
The main diagonal, also called the forward diagonal, or the major diagonal, is the set of diagonal matrix elements from upper left to lower right. The set of diagonal elements from lower left to upper right is of significantly less interest to us, but has several names including the antidiagonal, back diagonal, secondary diagonal, or the minor diagonal.
-
Identity Matrix
An identity matrix (
) is a square, diagonal matrix where all of the elements on the main diagonal are one. Identity matrices are like a one in scalar math. That is, the product of any matrix with the identity matrix yields itself.
MATLAB has a function called
eye
that takes one argument for the matrix size and returns an identity matrix.>> I2 = eye(2) I2 = 1 0 0 1 >> I3 = eye(3) I3 = 1 0 0 0 1 0 0 0 1
-
Upper Triangular Matrix
Upper triangular matrices have all zero values below the main diagonal. Any nonzero elements are on or above the main diagonal.
-
Lower Triangular Matrix
Lower triangular matrices have all zero values above the main diagonal. Any nonzero elements are on or below the main diagonal.
-
Symmetric Matrix
A symmetric matrix (
) has symmetry relative to the main diagonal. If the matrix where written on a piece of paper and you folded the paper along the main diagonal then the off-diagonal elements with the same value would lie on top of each other. For symmetric matrices
. Note that for any matrix,
, the products
and
both yield symmetric matrices. Here are a couple examples of symmetric matrices.
A symmetric matrix with complex elements is said to be Hermitian.
-
Orthonormal columns
A matrix,
, has orthonormal columns if the columns are unit vectors (length of 1) and the dot product between the columns is zero (
). That is to say, the columns are all orthogonal to each other.
-
Unitary Matrix
A matrix
is unitary if it is both orthonormal and square. The inverse of a unitary matrix is its conjugate (Hermitian) transpose,
. The product of these matrices with its Hermitian transpose is the identity matrix,
, and
.
-
Orthogonal Matrix
A matrix is orthogonal if its elements are real,
, and it is unitary.
Here is an example of multiplication of an orthogonal matrix by its transpose.
When we discuss the accuracy of solutions to systems of equations
() in Numerical Stability, we will
see that the condition number of the
matrix influences
the level of obtainable accuracy (Matrix Condition Number). Orthogonal
matrices have a condition number of 1, so Orthogonal Matrix Decompositions are used to
obtain the most accurate results, especially when
is
rectangular.
Note
Orthogonal matrices may seem a bit abstract at this point because we have not yet encountered an application for them. We need an orthogonal matrix to provide the basis vectors for vector projections, which is described in Alternate Projection Equation. Rotation matrices discussed in Rotation of a Point are orthogonal. We can obtain orthogonal columns from an existing matrix by using the modified Gram–Schmidt algorithm (Implementation of Modified Gram–Schmidt), QR factorization (QR Decomposition), or from the SVD (Finding Orthogonal Basis Vectors, and Projection and the Economy SVD). Orthogonal matrices are also the result from finding the eigenvectors of symmetric matrices, which is proven in The Schur Decomposition of Symmetric Matrices.
6.2.3. Special Matrix Relationships¶
6.2.3.1. Matrix Inverse¶
The inverse of a square matrix, , is another matrix,
, that multiplies with the original matrix to
yield the identity matrix.
Not all square matrices have an inverse and calculating the inverse for larger matrices is slow, requiring significant calculations.
In MATLAB, the function inv(A)
returns the inverse of matrix
. MATLAB’s left-divide operator provides a more efficient
and numerically stable alternative to using a matrix inverse to solve a
system of equations. So while matrix inverses frequently appear in
written documentation, we seldom calculate a matrix inverse.
Here are a couple properties for matrix inverses.
.
Start with the simple observation that
and
( Matrix Transpose Properties).
6.2.3.2. Matrix Transpose Properties¶
We described the transpose for vectors and matrices in Transpose.
The transpose with respect to addition,
.
. Notice that the order is reversed.
We often pre-multiply vectors and matrices by their transpose (
). The result is a scalar for a column vector, and a square, symmetric matrix for a row vector, rectangular matrix, and square matrix. If
is a
matrix,
is a
square, symmetric matrix.
Since
is symmetric, then
.
Another interesting result is that the trace of
, denoted as
, is the sum of the squares of all the elements of
. The trace of a matrix is the sum of its diagonal elements.
For an orthogonal matrix,
. This is called the Spectral Theorem. Note that the columns of the matrix must be unit vectors for this property to be true.
Since each of the columns are unit vectors, it follows that the diagonal of
is ones. The off-diagonal values are zeros because the dot product between the columns is zero. Thus,
Here is an example of an orthogonal rotation matrix.
>> Q Q = 0.5000 -0.8660 0.8660 0.5000 >> Q' ans = 0.5000 0.8660 -0.8660 0.5000 >> Q(:,1)'*Q(:,2) % column dot products => orthogonal ans = 0 >> Q'*Q % orthogonal columns ans = 1.0000 -0.0000 -0.0000 1.0000 >> Q*Q' % orthogonal rows ans = 1.0000 0.0000 0.0000 1.0000
Another interesting result about orthogonal matrices is that the product of two orthogonal matrices is also orthogonal. This is because both the inverse and transpose of matrices reverse the matrix order. Proof of orthogonality only requires that
.
Consider two orthogonal matrices
and
.
When a transpose is made of a matrix with complex values, we usually also take the complex conjugate of each value, which is called a Hermitian transpose. The conjugate of each value is often needed in numerical algorithms because when a complex number is multiplied by its conjugate, the product is a real number. The complex matrix product
is said to be Hermitian. Hermitian matrices have real numbers along the diagonal and the other values are symmetric conjugates. In technical writing, the Hermitian transpose is usually indicated as
. In some older publications, it may be written as
or even as
. Also in technical writing, symmetric matrices that may or may not have complex values are usually referred to as Hermitian rather than as symmetric.
In MATLAB,
A’
is the Hermitian transpose ofA
. WhileA.’
is the transpose ofA
where the complex conjugates of the values inA
are not taken.
6.2.4. Determinant¶
The determinant of a square matrix is a number. At one time, determinants were a significant component of linear algebra. But that is not so much the case today [STRANG99]. The importance of determinants is now more for analytical than computational purposes. Thus, while it is important to know what a determinant is, we will avoid using them when possible.
- Either of two notations are used to indicate the determinant of a
matrix,
or
.
- The classic technique for computing a determinant is called the
Laplace expansion. The complexity
of computing a determinant by the classic Laplace expansion method
has run-time complexity on the order of
(n–factorial), which is fine for small matrices, but is too slow for large (
) matrices.
- In practice, the determinant for matrices is computed using a faster
method that is described in Determinant Shortcut and improves the
performance to
. [3] The faster method uses the result that
. The LU decomposition factors are computed as described in LU Decomposition, then the determinant is the product of the determinants of the LU decomposition factors.
- In some applications, such as machine learning, very large matrices are often used, so computing determinants is impractical. Fortunately, we now have alternate algorithms that do not require determinants.
The determinant of a matrix is simple, but it gets
more complicated as the matrix dimension increases.
The determinant makes use of
determinants, called cofactors. In each stage
of Laplace expansion the elements in a row or column is multiplied by a
cofactor determinant from the other rows and columns excluding the row
and column of the multiplication element. The determinant is then the
sum of the expansion along the chosen row or column. But then the
determinants need to be calculated. Likewise
for the following smaller determinants until the needed determinant is a
that is solved directly. It works as follows for a
determinant.
Another approach to remembering the sum of products needed to compute a
determinant is to compute diagonal product terms. The
terms going from left to right are added while the right to left terms
are subtracted. Note: This only works for
determinants.
For a determinant, the pattern continues with each
cofactor term now being a
determinant. The pattern
likewise continues for higher order matrices. The number of computations
needed with this method of computing a determinant is on the order of
, which is prohibitive for large matrices.
Notice that the sign of the cofactor additions alternate according to the pattern:
It is not necessary to always use the top row for Laplace expansion. Any row or column will work, just make note of the signs of the cofactors. The best strategy is to apply Laplace expansion along the row or column with the most zeros.
Here is bigger determinant problem with lots of zeros so it is not too bad. At each stage of the expansion there is a row or column with only one nonzero element, so it is not necessary to find the sum of cofactor determinants. Trace the Laplace expansion as we find the determinant.
MATLAB has a function called det
that takes a square matrix as input
and returns the determinant.
6.2.5. Calculating a Matrix Inverse¶
The inverse of a square matrix, , is another matrix,
that multiplies with the original matrix to
yield the identity matrix.
Unfortunately, calculating the inverse of a matrix is computationally
slow. A formula known as Cramer’s Rule provides a neat and tidy
equation for the inverse, but for matrices beyond a ,
it is prohibitively slow. Cramer’s rule requires the calculation of the
determinant and the full set of cofactors for the matrix. Cofactors are
determinants of matrices one dimension less than the original matrix. To
find the inverse of a
matrix requires finding one
determinant and 9
determinants.
The run time complexity of Cramer’s rule is
.
For a matrix, Cramer’s rule can be found by algebraic
substitution.
One can perform the above matrix multiplication and find four simple equations from which the terms of the matrix inverse may be derived.
Because of the computational complexity, Cramer’s rule is not used by
MATLAB or similar software. Another technique known as elimination is
used. The elimination algorithm is descried in Elimination. The
run time complexity of calculating a matrix inverse by elimination is
. The good news is that we do not need to
manually calculate the inverse of matrices because MATLAB has a matrix
inverse function called
inv
.
>> A = [2 -3 0;4 -5 1;2 -1 -3];
>> A_inv = inv(A)
A_inv =
-1.6000 0.9000 0.3000
-1.4000 0.6000 0.2000
-0.6000 0.4000 -0.2000
Note
For square matrices, the left-divide operator uses an efficient elimination technique called LU decomposition to solve a system of equations. Thus, it is seldom necessary to compute the full inverse of the matrix.
6.2.6. Invertible Test¶
Not all matrices can be inverted. A matrix that is not invertible is said to be singular. A matrix is singular if some of its rows or columns are dependent on each other as described in Independent Vectors. An invertible matrix must derive from the same number of independent equations as the number of unknown variables. A square matrix from independent equations has rank equal to the dimension of the matrix, which means that it is full rank, has a nonzero determinant, and is invertible. We will define rank more completely after discussing elimination. For now, just think of rank as the number of independent rows and columns in the matrix. See also Systems of Linear Equations that describes how linear equations are mapped to matrix equations. A function described in The Column and Row Factorization shows which columns are independent and how the columns are combined to yield any dependent columns. Linearly Independent Vectors gives more formal definitions of independent and dependent vectors.
The following matrix is singular because column 3 is just column 1 multiplied by 2.
Sometimes it is difficult to observe that the rows or columns are not independent. When a matrix is singular, its rank is less than the dimension of the matrix, and its determinant also evaluates to zero.
>> A = [1 0 2;2 1 4;1 2 2];
>> rank(A)
ans =
2
>> det(A)
ans =
0
>> det(A') % the transpose is also singular
ans =
0
If you work much with MATLAB, you will occasionally run across a warning message saying that a matrix is close to singular. The message may also reference a rcondition number. The condition number and rcondition number are metrics indicating if a matrix is invertible, which we will describe in Matrix Condition Number. For our discussion here, we will focus on the rank as our invertible test.
6.2.7. Cross Product¶
The cross product is a special operation using two vectors in
with application to geometry and physical systems.
Although cross product is an operation for vectors, it is included here
because matrix operations (determinant or multiplication) is used to
compute it.
The cross product of two nonparallel 3-D vectors is a vector perpendicular to both vectors and the plane which they span and is called the normal vector to the plane. The direction of the normal vector follows the right hand rule as shown in figure Fig. 6.12. Finding the normal vector to a plane is needed to write the equation of a plane. Projections Onto a Hyperplane shows an example of using a cross product to find the equation of a plane.
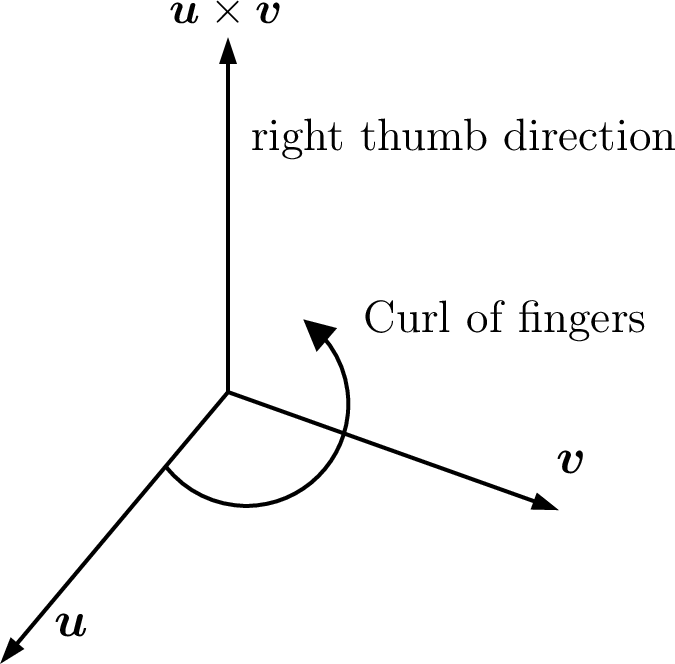
Fig. 6.12 Right Hand Rule: points in the
direction of your right thumb when the fingers curl from
to
.
is spoken as “
cross
”.
is perpendicular to both
and
.
is
.
- The length
, where
is the angle between
and
.
- The MATLAB function
cross(u, v)
returns.
-
Cross Product by Determinant
The cross product of
and
is a vector.
Vectors
,
, and
are the unit vectors in the directions of
,
, and
of the 3-D axis.
-
Cross Product by Skew-Symmetric Multiplication
An alternative way to compute
is by multiplication of a skew-symmetric, or anti-symmetric matrix.
The skew-symmetric matrix of
is given the math symbol,
. Such a matrix has a zero diagonal and is always singular. The transpose of a skew-symmetric matrix is equal to its negative.
MATLAB function
skew(u)
returns.
For vectors in
:
>> u = [1 2 3]'; >> v = [1 0 1]'; >> cross(u,v) ans = 2 2 -2 >> s = skew(u) s = 0 -3 2 3 0 -1 -2 1 0 >> s*v ans = 2 2 -2
Footnotes
[1] | The meaning of the SVD factors is not our concern at this point. In this application, it is only an example factoring. However, the SVD was chosen because the three factors have the interesting property of rotating, scaling, and then rotating points or vectors again. |
[2] | The factors of the SVD are ![]() ![]() ![]() |
[3] | The symbol ![]() ![]() ![]() ![]() ![]() ![]() |