6.8. Linear System Applications¶
Systems of linear equations are routinely solved using matrices in science and engineering. Here, two applications are reviewed.
6.8.1. DC Electric Circuit¶
A direct current (DC) electrical circuit with only a battery and resistors provides a system of linear equations. We want to determine the current and voltage drop across each resistor in the circuit. We only need Ohm’s Law and either Kirchhoff’s Voltage Law or Kirchhoff’s Current Law to find a solution.
Note
An AC circuit with capacitors and inductors in addition to resistors is described by a system of differential equations that can also be solved with linear algebra. MATLAB and linear algebra make determining such solutions straight forward; however, additional mathematical abstractions are needed, so we’ll stick with the simpler DC circuit with only resistors.
-
Ohm's Law
When a current of
amperes flows through a resistor of
ohms, the voltage drop,
, in volts across the resistor is
-
Kirchhoff's Voltage Law (KVL)
Each closed loop of a circuit is assigned a loop current. The current through resistors that are part of two loops is a combination of the loop currents. Then, the sum of the voltage drops around each loop is zero.
-
Kirchhoff's Current Law (KCL)
The sum of the currents entering and leaving each node of the circuit is zero. The voltages at each node of the circuit may be quantified in terms of any known voltages, voltage drops across resistors, and other node voltages.
Both the KVL and KCL methods lead directly to matrix equations.
6.8.1.1. KVL Method¶
The circuit is shown in Fig. 6.19 with the loop currents and their directions identified. The constant values used in the MATLAB program are: R1 = 1000; R2 = 1500; R3 = 2000; R4 = 1000; R5 = 1500; V = 12.
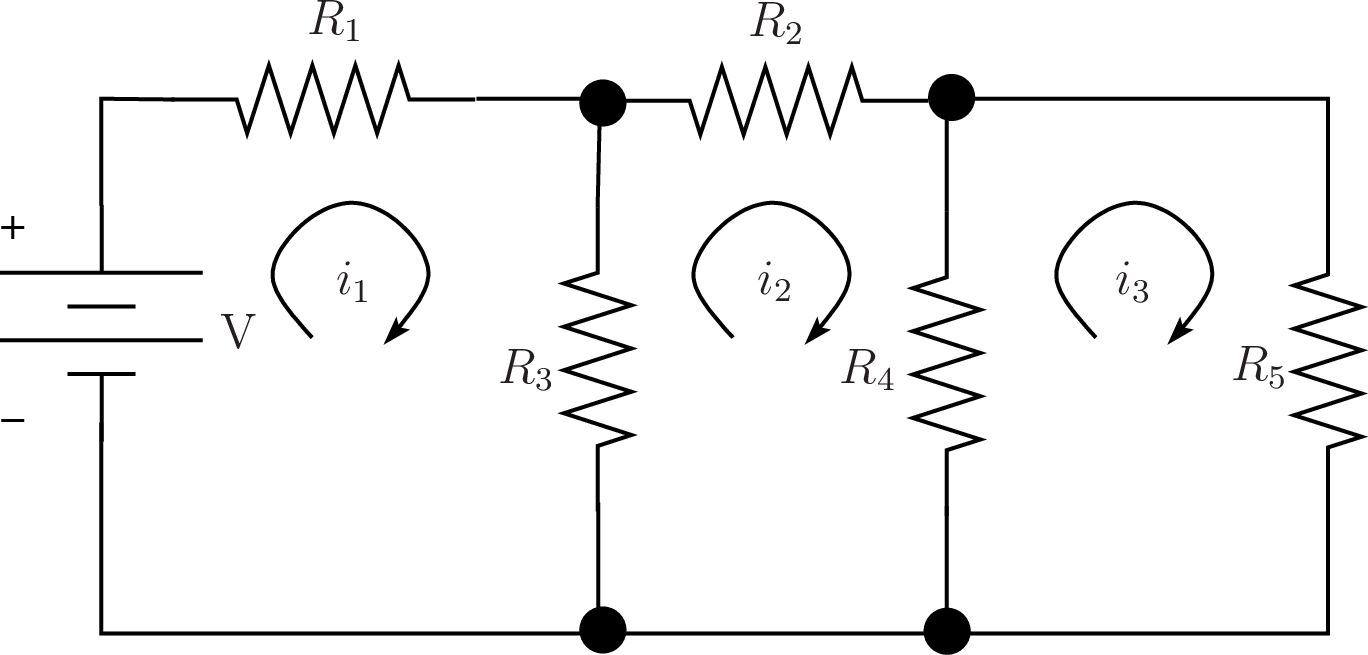
Fig. 6.19 DC circuit with loop currents identified for solving with the KVL method.
The three loop equations are given in terms of the loop currents.
6.8.1.2. KCL Method¶
With the KCL method, the sum of the currents entering or exiting each node must
equal zero. We only need to concern ourselves with the nodes labeled
and
in Fig. 6.20.
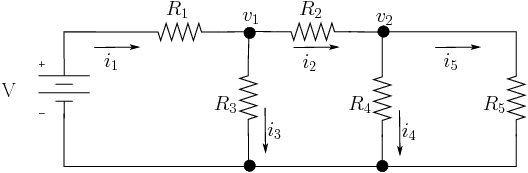
Fig. 6.20 DC circuit with node voltages and currents identified for solving with the KCL method.
Our system of equations is the currents entering and exiting nodes
labeled and
.
We use Ohm’s law to write each current in terms of the voltages across resistance values, which gives us sums of fractions.
To remove the fractions, multiply each equation by the products of the
denominators. Thus we multiply the terms of the first equation by
. The second equation is multiplied by
. Then we combine terms by the unknown node voltages
to make the matrix equations.
6.8.1.3. MATLAB Code¶
After doing the KVL calculation, the code computes some node voltages to help verify the correctness of the voltages from the KVL and KCL solutions.
%% Constants
R1 = 1000;
R2 = 1500;
R3 = 2000;
R4 = 1000;
R5 = 1500;
v = 12;
%% KVL Solution
R = [(R1+R3) -R3 0;
-R3 (R2+R3+R4) -R4;
0 -R4 (R4+R5)];
V = [v; 0; 0];
I = R \ V; % V = RI
i1 = I(1);
i2 = I(2);
i3 = I(3);
V1 = R1*i1;
V2 = R2*i2;
V3 = R3*(i1-i2);
V4 = R4*(i2-i3);
V5 = R5*i3;
fprintf('\n\tKVL Solution:\n')
fprintf('Current:\n i1 = %f\n i2 = %f\n i3 = %f\n',i1,i2,i3);
fprintf(...
'Voltages:\n V1 = %f\n V2 = %f\n V3 = %f\n V4 = %f\n V5 = %f\n', ...
V1,V2,V3,V4,V5);
disp('Verify: ')
fprintf(' %f == %f ?\n',V4,V5);
fprintf(' %f == %f ?\n',V3,(V2+V4));
fprintf(' %f == %f ?\n',v,(V3+V1));
%% KCL Solution
A = [(R2*R3 + R1*R3 + R1*R2) -R1*R3;
R4*R5 (-R4*R5 - R2*R5 - R2*R4)];
I = [R2*R3*v 0]';
V = A \ I; % (1/R)*V = I, A = 1/R -> AV = I
v1 = V(1);
v2 = V(2);
fprintf('\n\tKCL Solution:\n')
fprintf('Current:\n i1 = %f\n i2 = %f\n',(v-v1)/R1, (v1-v2)/R2);
fprintf(' i3 = %f\n i4 = %f\n i5 = %f\n',v1/R3, v2/R4, v2/R5);
fprintf('Voltages:\n V1 = %f\n V2 = %f\n ', v-v1, v1-v2);
fprintf('V3 = %f\n V4 = %f\n V5 = %f\n', v1, v2, v2);
This program displayed the following output. One can see that the KVL and
KCL methods gave the same results. Remember that the currents reported for
the KVL method are loop currents, not the currents through all of the
resistors. The current through , and
are combinations
of the loop currents.
KVL Solution:
Current:
i1 = 0.005928
i2 = 0.002892
i3 = 0.001157
Voltages:
V1 = 5.927711
V2 = 4.337349
V3 = 6.072289
V4 = 1.734940
V5 = 1.734940
Verify:
1.734940 == 1.734940 ?
6.072289 == 6.072289 ?
12.000000 == 12.000000 ?
KCL Solution:
Current:
i1 = 0.005928
i2 = 0.002892
i3 = 0.003036
i4 = 0.001735
i5 = 0.001157
Voltages:
V1 = 5.927711
V2 = 4.337349
V3 = 6.072289
V4 = 1.734940
V5 = 1.734940
6.8.2. The Statics of Trusses¶
The field of statics is concerned with determining the forces acting on members of stationary rigid bodies. Knowing the tension and compression forces are needed to ensure that the systems are designed to be strong enough. Here we consider a truss structure made of four members. Statics textbooks such as [MERIAM78] explain how to solve such systems.
Statics problems such as shown in Fig. 6.21 are solved at two levels. First, we consider the external forces on the overall structure, which are later applied to the members of the structure. Three equations are used for this step.
Sum of the moments is zero. Here we define a focal point and then calculate the moment of each external force as the product of the force value perpendicular to the structure times distance from the focal point. This ensures that the structure does not rotate. Note that in the problem that we solve here, the tension force of the
member is not perpendicular to the structure, so the cosine function is used to project a perpendicular force.
Sum of forces in the
direction is zero.
Sum of forces in the
direction is zero.
Secondly, the method of joints is used to determine the force on each member.
We examine each joint and write equations for the forces in the
and the
directions. These forces must also sum to zero for each
joint.
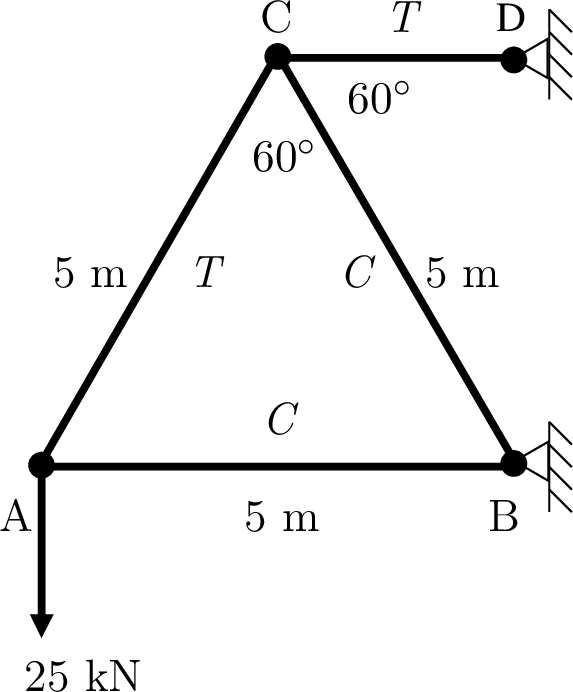
Fig. 6.21 A static truss based support. We need to determine the tension or compression force on each member of the truss. Each member is marked with a T or C as either bearing a tension or compression force.
As you can see from Fig. 6.21, this is a fairly simple problem – 4
members between 3 joints and the external supports. As a full system of
equations, there are 9 equations – 3 external force equations and 2 equations
each for the 3 joints: ,
, and
. When taken in the
desired order, each algebra equation reduces to having only one unknown
variable. We’ll take a middle path between an over-determined system of 9
equations with 4 unknowns and a carefully ordered sequence of algebra problems.
We list the 9 equations and then pick 4 equations for a
matrix equation. Three of picked equations have only one unknown variable. The
fourth equation has two unknown variables. The 9 equations are listed below.
The numbered equations are selected for used in the matrix solution.
- [1 – Sum of moments:
]
- [External forces:
]
- [External forces:
]
- [2 – A:
]
- [3 – A:
]
- [B:
]
- [4 – B:
]
- [C:
]
- [C:
]
The rows of the A matrix are ordered such that it is upper triangular, which the left-divide operator will take advantage of.
% File: truss.m
% Find the forces on truss members in figure 5.21.
c60 = cosd(60);
c30 = cosd(30);
s30 = sind(30);
A = [1 -c60 0 0; % rows ordered for triangular structure
0 c30 0 0;
0 0 c30 0;
0 0 0 c30];
b = [0; 25; 25; 25];
x = A\b;
fprintf('AB: %g kN C\n', x(1));
fprintf('AC: %g kN T\n', x(2));
fprintf('BC: %g kN C\n', x(3));
fprintf('CD: %g kN T\n', x(4));
The forces on each member are found to the following.
>> truss
AB: 14.4338 kN C
AC: 28.8675 kN T
BC: 28.8675 kN C
CD: 28.8675 kN T