6.3.2. Homogeneous Matrix¶
Geometric translation is often added to the rotation matrix to make a matrix
that is called the homogeneous transformation matrix. The translation
coordinates ( and
) are added in a third column. A third
row is also added so that the resulting matrix is square, .
Homogeneous rotation alone is given by the matrix.
Homogeneous translation alone is given by the matrix.
The combined rotation and translation homogeneous transformation matrix can be found by matrix multiplication.
6.3.3. Applying Transformations to a Point¶
When applied to a point, the homogeneous transformation matrix uses rotation followed by translation in the original coordinate frame. It is not translation followed by rotation.
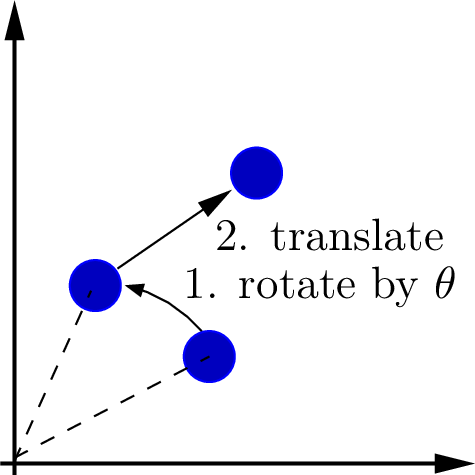
Fig. 6.13 A transformation applied to a point, , rotates and translates the point relative
to the coordinate frame.¶
In the following MATLAB session, we define matrix and translation matrices using homogeneous coordinates. We compound the two with matrix multiplication and apply the compound transformation matrix to a point. The rotation and translation matrices are constructed with a sequence of commands. Peter Corke’s Spatial Math Toolbox provides functions that expedite the task. Depending on your interests, you might also be interested in his toolboxes for robotics and machine vision.
>> % Rotation matrix
>> theta = pi/3;
>> R2 = [cos(theta) -sin(theta); sin(theta) cos(theta)]
R2 =
0.5000 -0.8660
0.8660 0.5000
>> R = [R2 [0; 0]; [0 0 1]] % homogeneous coordinates
R =
0.5000 -0.8660 0
0.8660 0.5000 0
0 0 1.0000
>> % translation matrix
>> xt = 2; yt = 1;
>> tr = eye(3); tr(1:2,3) = [xt; yt]
tr =
1 0 2
0 1 1
0 0 1
>> % transformation matrix, rotation (pi/3) and translation (2, 1)
>> T = tr*R
T =
0.5000 -0.8660 2.0000
0.8660 0.5000 1.0000
0 0 1.0000
>> % Define a point at (1, 1)
>> p = [1;1]; % Cartesian coordinates
>> p1 = [p;1] % Homogeneous coordinates
p1 =
1
1
1
>> % transformation of p
>> p2 = T*p1
p2 =
1.6340
2.3660
1.0000
>> p_new = p2(1:2) % Cartesian coordinates
p_new =
1.6340
2.3660
We can also apply the transformation in Cartesian coordinates, but it is not a simple matrix multiplication as it is in homogeneous coordinates. We rotate the point and then add the translation to the coordinates.
>> R2*p + [xt; yt]
ans =
1.6340
2.3660