11.3. Homework 3 - Control Constructs and Newton’s Square Root Algorithm¶
In class, we discussed an algorithm developed by Sir Isaac Newton to
find the square root of a number using an iterative approximation algorithm.
We worked an example of how to implement the algorithm using a for
loop. For this assignment, you should replace the for
loop with a
while
loop, which determines if the result after each loop iteration
is close enough to the square root of . Note that there are multiple
ways to determine this. You could square the result and compare it to K. You
could also check for convergence of the result because once the
is found, further loop iterations will not change the result.
Report how many times the loop executed to calculate the answer.
Numeric Roots of a Function Algorithm
The algorithm for finding the square root of a number is actually an instance
of an algorithm for finding a root of a function ( for which
).
The Newton-Raphson method, or just Newton’s method, uses lines that are tangent to the curve of a function to estimate where the function crosses the x-axis.
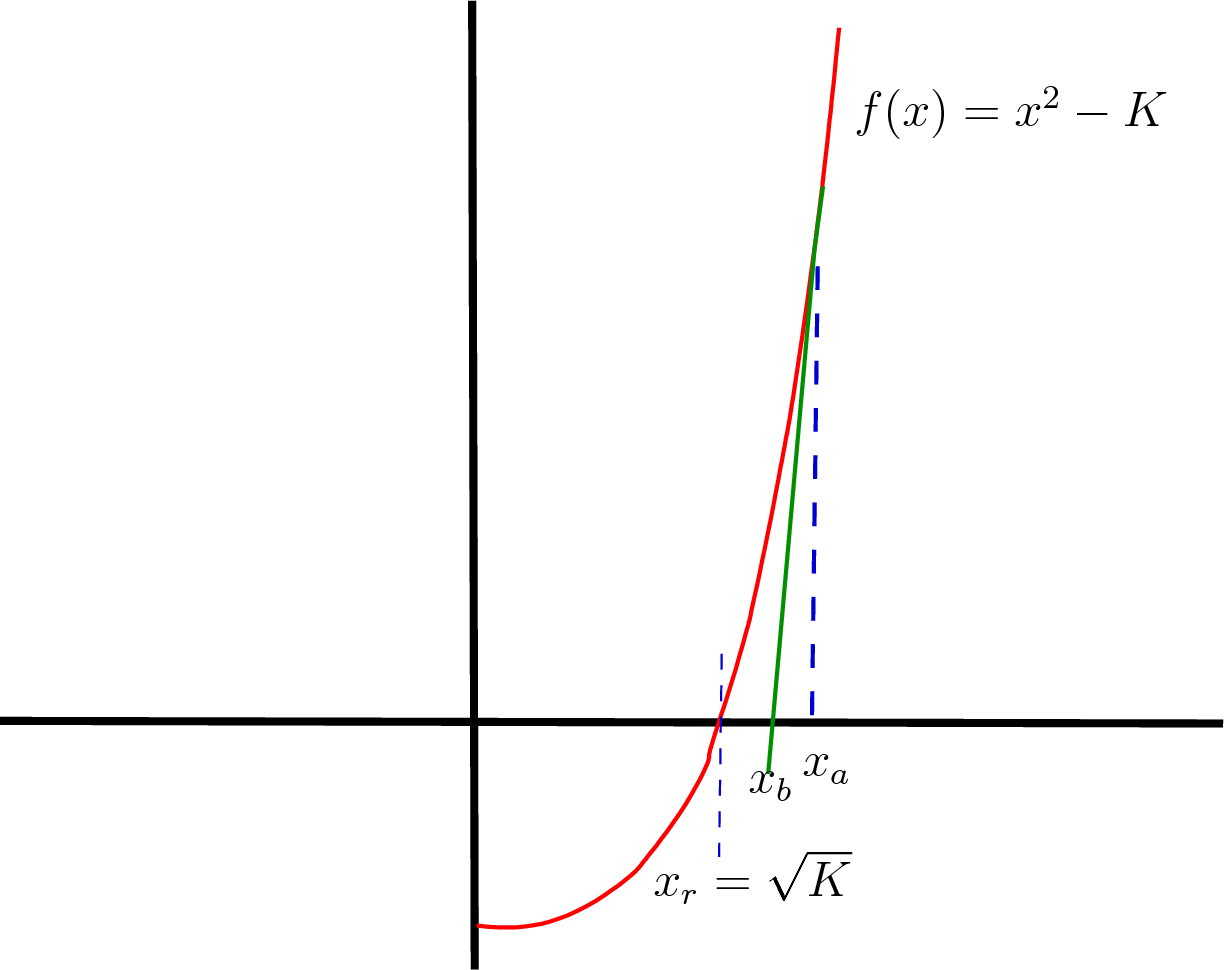
Fig. 11.1 The Newton-Raphson method extends a tangent line to the x-axis to estimate of a root and repeats the process until the root is found with an allowed tolerance.
The algorithm evaluates the function at a sequence of estimates until the root is found.
Evaluate the function and its derivative at the first estimate,
and
.
The equation for a tangent line passing through the x-axis is
The estimate for the root is then
If
is not close enough to the root, let
and repeat the steps.
The above graph shows a function for finding . Let
, then
.
Newton’s Square Root Algorithm
For any , we wish to calculate the exact value or a
close approximation to
.
Begin with any ,
. We define the next
approximation to
,
, as:
If we define the errors between the actual square root of
and
and
as
and
,
then Newton’s Square Root Algorithm claims that:
That is either, or
is
closer to the value of
than is
.
If :
and
.
If :
and
, which is the average of
and
, will be greater than
and thus closer to
than
.
If :
and
, which is the average of
and
, will be less than
and thus closer to
than
.
It follows then that a loop may be constructed to approximate
:
x_a = x/2;
loop:
x_b = (x_a + K/x_a)/2;
x_a = x_b;
The matter of how many times to execute this loop is the primary concern of this homework assignment.